I recently covered FreeShow, a software made for presentations in Churches. One of the features that I liked and talked about was the open-source nature of the App. In this case, open-source means having the app’s code publicly available on GitHub, with any changes made, issues, or additions to its Codebase available to read / post.
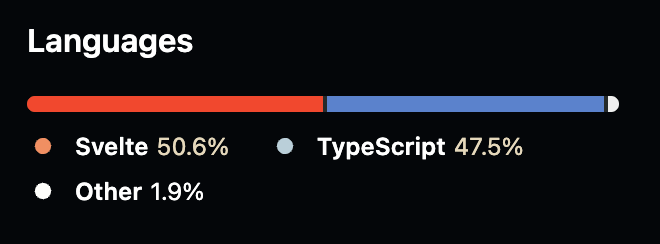
I noticed while poking around the GitHub page for FreeShow, is a large percentage of the code’s language is written in TypeScript.5
Typescript is similar to Javascript in that it adds to the existing framework of JS with additional checks like types, which ensures the data of a variable is the correct “type” it’s been told it is, making it easier to catch errors. I’ve been learning Javascript through Coursera’s Front-end Web Development course, and even though not explicitly teaching TypeScript, Typescript contains everything Javascript has, just with additional features, making it a more straightforward matter of learning those additional features rather than a whole new language.
With the knowledge of FreeShow’s codebase being primarily of a language I could understand, I decided to fork the repository (forking creates a copy of the repository, which is separated from the main code). I started looking through the code to see how it’s constructed and if I could implement my features into the app, that would be useful.
I also later found this page, which contains all the info they give to devs willing to contribute, like where they need help with apps and how their code is typically structured.
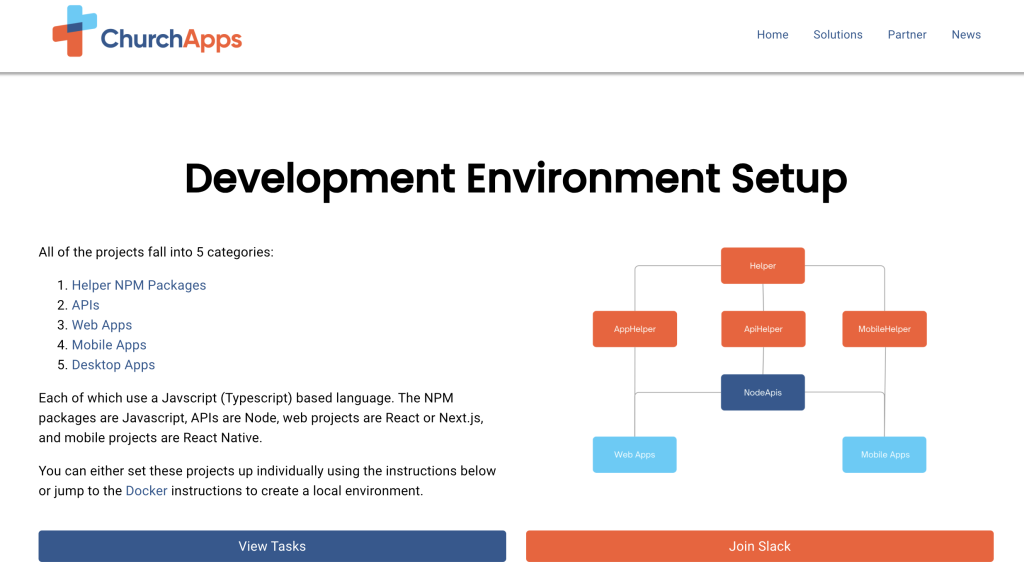
I started with one implementation I would find helpful: a way for the application to track when a song gets “used” and then, with that information, have it pull from the oldest used songs from the current date. The primary use case for this feature would be picking songs for Sunday’s service. The current way we do it with Google Slides is to attach an ID to each slideshow, and as the year goes by, eventually, it adds up to #100. In which case, we start over with Sunday #001’s songs. Making it impossible to select a song we sang last week.
Luckily, FreeShow already has a similar variable implemented to track when a song has been last used, called “used.” With that, I made a simple helper script that attaches to the existing “used” variable, “Chronicle.” Chronicle compares the used date with a reference date like Jan 01, 2024, and returns the day value of that comparison.
export function chronicleShow(timestamp: number) {
const usedDate: number = new Date(timestamp).getTime();
const chronicleRef: number = new Date('2024-01-01').getTime();
const daysDifference = Math.floor((usedDate - chronicleRef) / (1000 * 60 * 60 * 24));
//output example "044" (44th day from chronicleRef)
return daysDifference.toString().padStart(3, '0');
}
In reality, this is a super simple script and hardly took any time, mostly being “inspired” by this post by Javatpoint. The more interesting part of adding a simple feature like this is digging through a full app’s code and figuring out what function is used for what. For example, finding where the “used” cache was getting updated was initially difficult. Still, eventually, I found it in a function called “update” because of a message that gets logged when opening a “show” item, and that message linked to the start of the trial to find the “used” cache update line.

Leave a Reply