A new concept I’m starting to learn in my Coursera course on React Basics (view post series here) is React “States.” States in React is an interesting way of storing the “state” or current information about a variable or function.
ⓘ As a new topic, I’m still learning how React works. For an actual professional, please refer to a better explanation.
The first example of utilizing state is the “React.useState” hook. To use useState, you assign it to two array values that usually consist of one variable storing the value and another variable to set the value of the state. For example:
import { useState } from 'react';
function exampleApp() {
const [user, setUser] = useState("ezra");
}
First, we import the state hook. Then, in the function, we assign two variables to the useState hook. Inside of the state, it has “Ezra,” which, in this case, sets the default value. If we wanted to add a function to update the state to a value, we could do so as follows:
function updateUser(e) {
setUser(e.target.value);
}
Coursera outlines that you generally want to utilize separate functions to handle events. In this case, we have a function that, when called in combination with an input field, will grab the input’s value (e.target.value). We use the setUser that we already declared earlier.
The complete component example they taught me was:
import { useState } from 'react';
function ExampleApp() {
const [user, setUser] = useState("ezra");
function updateUser(e) {
setUser(e.target.value);
}
return (
<div>
<h1>{user}</h1>
<input onInput={updateUser}></input>
</div>
)
}
export default ExampleApp;
This creates a page with an H1 element and an Input field. By default, H1 says “Ezra,” but when you type into the input field, it actively changes to what you type.
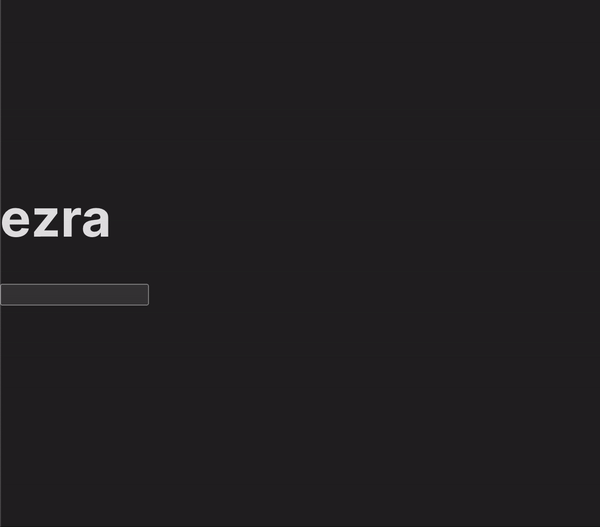
It is an excellent and useful concept that will probably be used frequently in future React programming. Coursera also outlined a general guideline for creating components, specifically when and when not to use states.
As the name implies, you have Stateless and Stateful components, one without any state declarations and others with state declarations. It goes to the basics of how you want things formatted and makes it much easier to later grab the state of an object by passing it down from a parent to a child component rather than having to reinstate that state in each element.
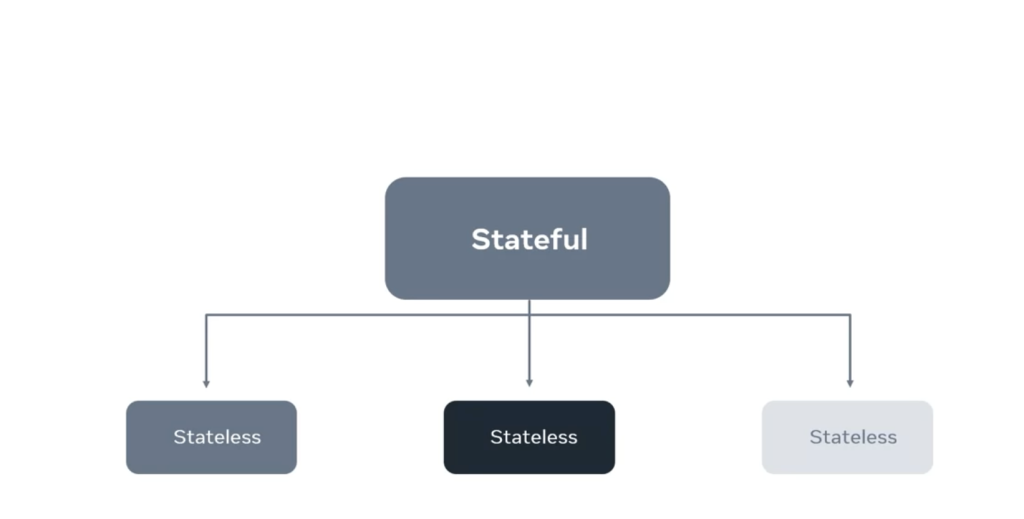
States in react go a lot deeper and a lot more complex, but I’m excited to learn more about it.